Skype using java API (Skype4Java)
demonstrate message sending feature.

Koji Hisano <hisano@gmail.com>, eflow Inc. and Skype Technologies S.A. have the copyright of Skype API for Java. Please, use this in user's self-responsibility.

First step in configuration is to download the Skype4Java API. To download the library, Click here
- Extract the compressed file(skype_1.0.zip) into your local hard drive.
- Check the directory namely release under skype directory and append the absolute path of the release directory to PATH environmental variable. [MS Windows]
- skype.jar and swt.jar of release directory should be placed under CLASSPATH environment variable.
Now, Start your Skype and log in using valid user name and password.

This sample java class will illustrate you the usage of ContactList and Friends classes. I have written this class for displaying all friends Id from my Skype contact list.
package com.sarf.skype4java; import com.skype.ContactList; import com.skype.Friend; import com.skype.Skype; import com.skype.SkypeException; public class ContactLister { public void getAllFriend() throws SkypeException, InterruptedException { //Getting all the contact list for log in Skype ContactList list = Skype.getContactList(); Friend fr[] = list.getAllFriends(); //Printing the no of friends Skype have System.out.println(fr.length); //Iterating through friends list for(int i=0; i < fr.length; i++) { Friend f = fr[i]; //Getting the friend ID System.out.println("Friend ID :"+f.getId()); Thread.sleep(100); } } public static void main(String[] args) t throws SkypeException, InterruptedException { ContactLister objCL = new ContactLister(); objCL.getAllFriend(); } }
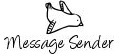
We can also send message to the people using a simple java class. The below excerpt is for sending message to the people in your contact list.
package com.sarf.skype4java; import com.skype.ContactList; import com.skype.Friend; import com.skype.Skype; import com.skype.SkypeException; public class MessageSender { public void getAllFriend() throws SkypeException, InterruptedException { //Getting all the contact list for log in Skype ContactList list = Skype.getContactList(); Friend fr[] = list.getAllFriends(); //Printing the no of contacts of your skype System.out.println(fr.length); //Iterating through friends list for(int i=0; i < fr.length; i++) { Friend f = fr[i]; // Sending message here f.send("Good evening "+f.getId()); Thread.sleep(100); } } public static void main(String[] args) throws SkypeException, InterruptedException { MessageSender objCL = new MessageSender(); objCL.getAllFriend(); } }

A common dialog window or a message dialog in your Skype application window will be opened asking you to give permission as shown below:
Select Allow this program to use skype click on OK button.
<< Home